Arduino-Trigonometry関数(三角関数)の使い方
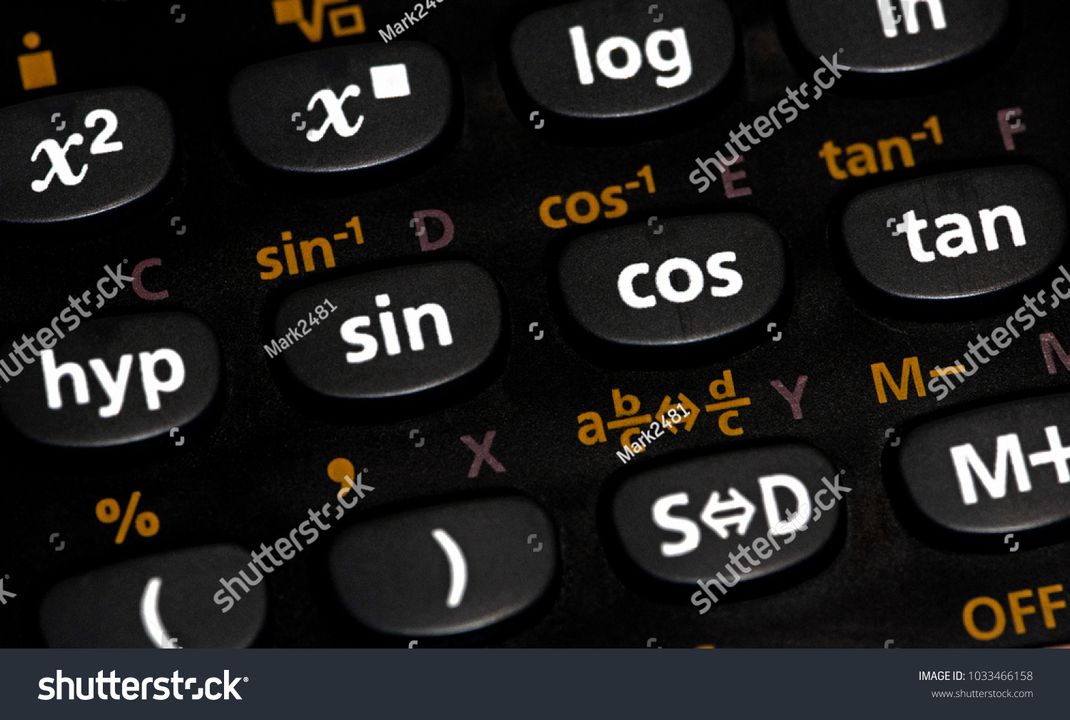
当記事では、ArduinoのTrigonometry関数(三角関数)の使い方について詳しく解説します。
Trigonometry関数を使うことによって、cos、sin、tanの計算をすることができます。
なお、その他のArduino関数・ライブラリについては、以下の記事をご覧ください。
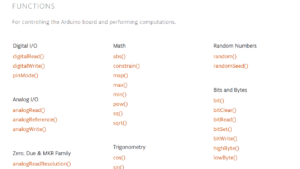
Trigonometry関数(三角関数)
関数 | 引数 | 戻り値 | 説明 |
---|---|---|---|
double cos(float rad) | rad:数値(rad) | cosで計算した値を返す | cos計算 |
double sin(float rad) | rad:数値(rad) | sinで計算した値を返す | sin計算 |
double tan(float rad) | rad:数値(rad) | tanで計算した値を返す | tan計算 |
cos()/cos計算
- 関数:double cos(float rad)
- 引数:rad⇒数値(rad)
- 戻り値:cosで計算した値を返す
cos()関数は、引数で指定した数値をcos計算(余弦計算)した値を戻り値で返します。
sin()/sin計算
- 関数:double sin(float rad)
- 引数:rad⇒数値(rad)
- 戻り値:sinで計算した値を返す
sin()関数は、引数で指定した数値をsin計算(正弦計算)した値を戻り値で返します。
tan()/tan計算
- 関数:double tan(float rad)
- 引数:rad⇒数値(rad)
- 戻り値:tanで計算した値を返す
tan()関数は、引数で指定した数値をtan計算(正接計算)した値を戻り値で返します。
サンプルプログラム(サンプルスケッチ)
- Arduino Uno
- USBケーブル
- PC(プログラム書き込み・シリアルモニタ表示)
cos()/cos計算
void setup() {
Serial.begin(9600);//シリアル通信を9600bpsで初期化
}
void loop() {
double cal;//「cal」をdoubleで変数宣言
cal = cos(0);//cos0(cos0°)を計算、1.00をcalに代入
Serial.print("cos0: ");//文字列「cos0: 」を送信
Serial.println(cal);//数字「1.00」を送信、改行
cal = cos(PI/2);//cosπ/2(cos90°)を計算、-0.00をcalに代入
Serial.print("cosπ/2: ");//文字列「cosπ/2: 」を送信
Serial.println(cal);//数字「-0.00」を送信、改行
cal = cos(PI);//cosπ(cos180°)を計算、-1.00をcalに代入
Serial.print("cosπ: ");//文字列「cosπ: 」を送信
Serial.println(cal);//数字「-1.00」を送信、改行
cal = cos(PI*3/2);//cos3π/2(cos270°)を計算、0.00をcalに代入
Serial.print("cos3π/2: ");//文字列「cos3π/2: 」を送信
Serial.println(cal);//数字「0.00」を送信、改行
cal = cos(PI*2);//cos2π(cos360°)を計算、1.00をcalに代入
Serial.print("cos2π: ");//文字列「cos2π: 」を送信
Serial.println(cal);//数字「1.00」を送信、改行
delay(1000);//1秒待機(1000msec待機)
}
cos0: 1.00
cosπ/2: -0.00
cosπ: -1.00
cos3π/2: 0.00
cos2π: 1.00
cos0: 1.00
cosπ/2: -0.00
cosπ: -1.00
cos3π/2: 0.00
cos2π: 1.00
サンプルプログラムは、cos0、cosπ/2、cosπ、cos3π/2、cos2πをそれぞれ計算して、シリアルモニタに表示します。cos()の引数は、角度ではなくrad単位になるので注意してください。
sin()/sin計算
void setup() {
Serial.begin(9600);//シリアル通信を9600bpsで初期化
}
void loop() {
double cal;//「cal」をdoubleで変数宣言
cal = sin(0);//sin0(sin0°)を計算、0.00をcalに代入
Serial.print("sin0: ");//文字列「sin0: 」を送信
Serial.println(cal);//数字「0.00」を送信、改行
cal = sin(PI/2);//sinπ/2(sin90°)を計算、1.00をcalに代入
Serial.print("sinπ/2: ");//文字列「sinπ/2: 」を送信
Serial.println(cal);//数字「1.00」を送信、改行
cal = sin(PI);//sinπ(sin180°)を計算、-0.00をcalに代入
Serial.print("sinπ: ");//文字列「sinπ: 」を送信
Serial.println(cal);//数字「-0.00」を送信、改行
cal = sin(PI*3/2);//sin3π/2(sin270°)を計算、-1.00をcalに代入
Serial.print("sin3π/2: ");//文字列「sin3π/2: 」を送信
Serial.println(cal);//数字「-1.00」を送信、改行
cal = sin(PI*2);//sin2π(sin360°)を計算、0.00をcalに代入
Serial.print("sin2π: ");//文字列「sin2π: 」を送信
Serial.println(cal);//数字「0.00」を送信、改行
delay(1000);//1秒待機(1000msec待機)
}
sin0: 0.00
sinπ/2: 1.00
sinπ: -0.00
sin3π/2: -1.00
sin2π: 0.00
sin0: 0.00
sinπ/2: 1.00
sinπ: -0.00
sin3π/2: -1.00
sin2π: 0.00
サンプルプログラムは、sin0、sinπ/2、sinπ、sin3π/2、sin2πをそれぞれ計算して、シリアルモニタに表示します。sin()の引数は、角度ではなくrad単位になるので注意してください。
tan()/tan計算
void setup() {
Serial.begin(9600);//シリアル通信を9600bpsで初期化
}
void loop() {
double cal;//「cal」をdoubleで変数宣言
cal = tan(0);//tan0(tan0°)を計算、0.00をcalに代入
Serial.print("tan0: ");//文字列「tan0: 」を送信
Serial.println(cal);//数字「0.00」を送信、改行
cal = tan(PI/2);//tanπ/2(tan90°)を計算、-22877332.00をcalに代入
Serial.print("tanπ/2: ");//文字列「tanπ/2: 」を送信
Serial.println(cal);//数字「-22877332.00」を送信、改行
cal = tan(PI);//tanπ(tan180°)を計算、0.00をcalに代入
Serial.print("tanπ: ");//文字列「tanπ: 」を送信
Serial.println(cal);//数字「0.00」を送信、改行
cal = tan(PI*3/2);//tan3π/2(tan270°)を計算、-83858280.00をcalに代入
Serial.print("tan3π/2: ");//文字列「tan3π/2: 」を送信
Serial.println(cal);//数字「-83858280.00」を送信、改行
cal = tan(PI*2);//tan2π(tan360°)を計算、0.00をcalに代入
Serial.print("tan2π: ");//文字列「tan2π: 」を送信
Serial.println(cal);//数字「0.00」を送信、改行
delay(1000);//1秒待機(1000msec待機)
}
tan0: 0.00
tanπ/2: -22877332.00
tanπ: 0.00
tan3π/2: -83858280.00
tan2π: 0.00
tan0: 0.00
tanπ/2: -22877332.00
tanπ: 0.00
tan3π/2: -83858280.00
tan2π: 0.00
サンプルプログラムは、tan0、tanπ/2、tanπ、tan3π/2、tan2πをそれぞれ計算して、シリアルモニタに表示します。tan()の引数は、角度ではなくrad単位になるので注意してください。
おすすめのArduinoボードはどれ?
当記事『Arduino-Trigonometry関数(三角関数)の使い方』では、Arduino Unoを使用したサンプルプログラムを解説してきました。
やはり、たくさんの種類のあるArduinoボードの中でも、最も基本的なエディションのArduino Unoがおすすめなのですが、Arduino Unoと電子部品を組み合わせたキットも存在します。
電子工作初心者にとっては、いちいち電子部品を別途購入する必要がないので非常に有用です。以下の記事で初心者でもわかりやすいように、ランキング形式でおすすめのArduino Unoを紹介しているので、ぜひご覧ください。
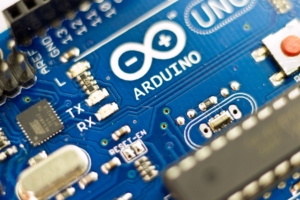
また、以下の記事で、安価でWi-Fi/Bluetoothに対応している「ESP32開発ボード」についてもまとめてみました。
このボードは、Arduinoボードではありませんが、Arduino IDEでソフト開発ができるため、電子工作でIoTを実現したい方におすすめです。
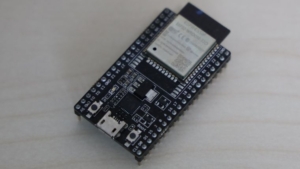